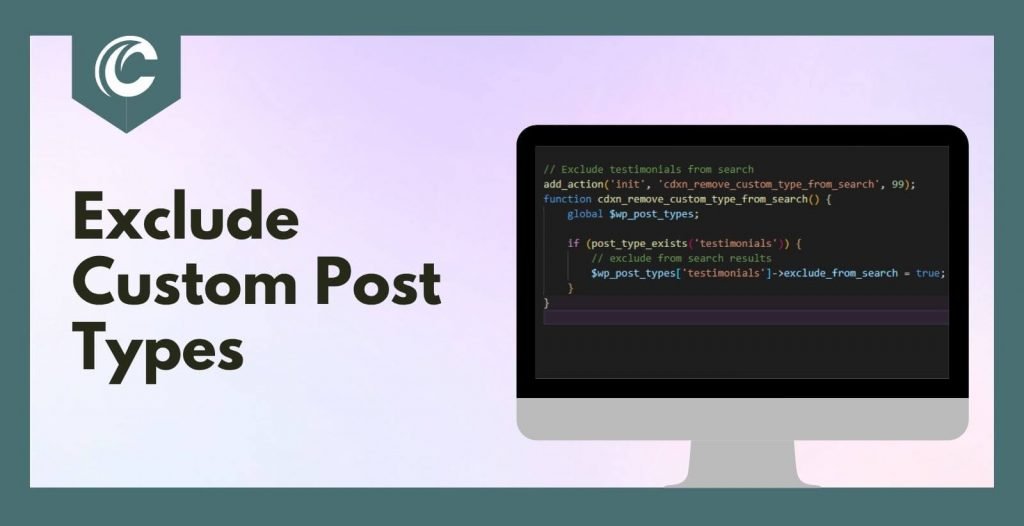
Sometimes, you may want to exclude custom post types from the WordPress search result page by using an easy and efficient way. Let’s consider two possible scenarios like below:
- You want to exclude custom post types from the WordPress search results at the time you create and register them, or
- You already have custom post types created somewhere in your WordPress theme or plugin files. And you don’t want to mess up with the original codes but still want to exclude them from the WordPress search result page.
Let’s discuss both of these scenarios one by one.
Exclude custom post type at the time you create and register the post type
In this example, we are going to create a custom post type named “Testimonials”. The related code for this will look like below:
// Register Custom Post Type - Testimonials and exclude from search result
add_action( 'init', 'cdxn_testimonials_cpt' );
function cdxn_testimonials_cpt() {
$labels = array(
'name' => _x( 'Testimonials', 'Testimonials', 'codexin' ),
'singular_name' => _x( 'Testimonial', 'Testimonials', 'codexin' ),
'menu_name' => __( 'Testimonials', 'codexin' ),
'parent_item_colon' => __( 'Parent Testimonial:', 'codexin' ),
'all_items' => __( 'All Testimonials', 'codexin' ),
'add_new_item' => __( 'Add New Testimonial', 'codexin' ),
'add_new' => __( 'Add New', 'codexin' ),
'new_item' => __( 'New Testimonial', 'codexin' ),
'edit_item' => __( 'Edit Testimonial', 'codexin' ),
'update_item' => __( 'Update Testimonial', 'codexin' ),
'view_item' => __( 'View Testimonial', 'codexin' ),
'view_items' => __( 'View Testimonials', 'codexin' ),
'search_items' => __( 'Search Testimonial', 'codexin' ),
'not_found' => __( 'Not found', 'codexin' ),
'not_found_in_trash' => __( 'Not found in Trash', 'codexin' ),
);
$args = array(
'label' => __( 'Testimonials', 'codexin' ),
'labels' => $labels,
'supports' => array(
'title',
'editor',
'thumbnail'
),
'hierarchical' => false,
'public' => true,
'show_ui' => true,
'show_in_menu' => true,
'menu_position' => 5,
'show_in_admin_bar' => true,
'show_in_nav_menus' => true,
'has_archive' => true,
'exclude_from_search' => true,
'publicly_queryable' => true,
'capability_type' => 'post',
'query_var' => true,
'rewrite' => true,
'menu_icon' => 'dashicons-art',
);
register_post_type( 'testimonials', $args );
}
Notice the $args
parameter with 'exclude_from_search' => true
. We have set the value for this parameter as true
. And thats it. Place the above code in yout theme’s functions.php
file. Once your custom post type is LIVE, they will be excluded automatically from the WordPress search result page.
Exclude custom post type from WordPress search for the already registered post types
For this case, I’m going to assume that you already have a custom post type registered inside your theme or plugin. And now you want to exclude them from the WordPress search result page so people don’t find them out on the WordPress search result page. Copy and paste the below code in your theme’s functions.php
file and you are all set.
// Exclude testimonials from search
add_action('init', 'cdxn_remove_custom_type_from_search', 99);
function cdxn_remove_custom_type_from_search() {
global $wp_post_types;
if (post_type_exists('testimonials')) {
// exclude from search results
$wp_post_types['testimonials']->exclude_from_search = true;
}
}
As you can see, we have set the same parameter exclude_from_search
as true
. And, finally hooked the function with WordPress init
hook with a priority of 99. You may need to replce the custom post type named ‘testimonials’ by your own.
Thank you for reading this article! We hope that it has been interesting and informative. You are more than welcome to visit our other blog posts on WordPress from here
Hi, thank you for this. I used your example for a pre-existing post type on my site and it worked great. How would you expand this to enable the exclusion of more than one custom post type? I tried the very basic approach of taking the content of the IF statement and adding an ELSEIF below for the second post type but this did not work.