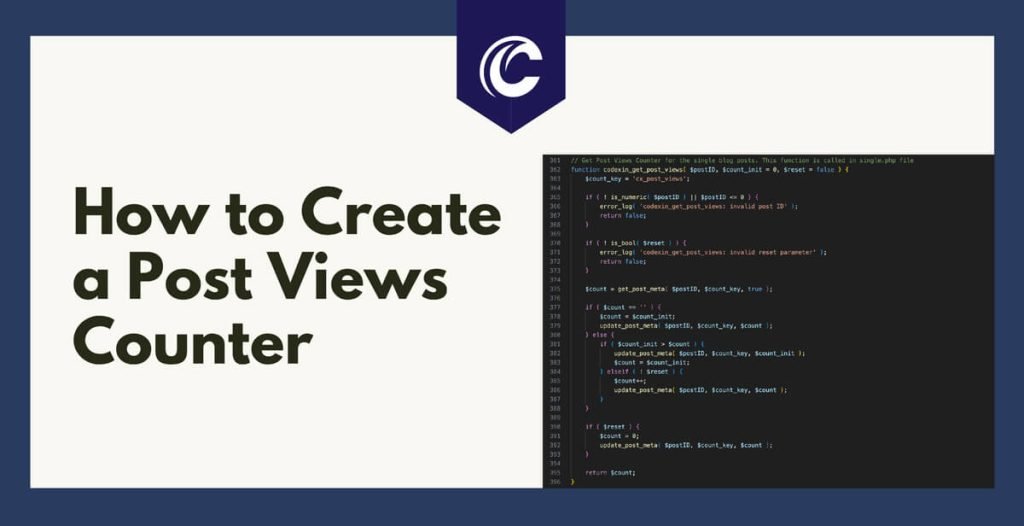
Having a post views counter in WordPress is a valuable feature to gauge the popularity of your blog posts. It provides quantitative data about reader engagement and can be particularly useful for websites with a broad array of content. Here’s how to create such a counter in WordPress. Additionally, learn how to add it as a custom column inside the WordPress dashboard All Posts page
Step 1: Creating a Post Views Counter
The views counter function is essentially a piece of code that increments the views count each time a visitor views a post. This function uses WordPress’s get_post_meta
and update_post_meta
functions to fetch and update the count, respectively.
To add this counter function, you would need to insert it into your theme’s functions.php file. For a more comprehensive solution, you might want to create a custom plugin and place the function there. Here is the function that will manage the post view counts:
// Get Post Views Counter for the single blog posts. This function is called in single.php file
function codexin_get_post_views( $postID, $count_init = 0, $reset = false ) {
$count_key = 'cx_post_views';
if ( ! is_numeric( $postID ) || $postID <= 0 ) {
error_log( 'codexin_get_post_views: invalid post ID' );
return false;
}
if ( ! is_bool( $reset ) ) {
error_log( 'codexin_get_post_views: invalid reset parameter' );
return false;
}
$count = get_post_meta( $postID, $count_key, true );
if ( $count == '' ) {
$count = $count_init;
update_post_meta( $postID, $count_key, $count );
} else {
if ( $count_init > $count ) {
update_post_meta( $postID, $count_key, $count_init );
$count = $count_init;
} elseif ( ! $reset ) {
$count++;
update_post_meta( $postID, $count_key, $count );
}
}
if ( $reset ) {
$count = 0;
update_post_meta( $postID, $count_key, $count );
}
return $count;
}
Each time a post is viewed, this function increments the view count.
Step 2: Displaying the Counter on Your Blog Posts
After setting up the function, you can call this function in your single.php
file to display the number of views each post has garnered. Here is an example of how you can do this:
// Displaying the number of post views inside single.php file
if ( have_posts() ) : while ( have_posts() ) : the_post();
// Your other code...
// Display the view count
$view_count = codexin_get_post_views( get_the_ID() );
printf( esc_html__( 'Visited %s times', 'text-domain' ), $view_count );
// Your other code...
endwhile; endif;
This piece of code will output a phrase like “Visited XXX times”, where XXX is the number of views.
Step 3: Adding the Post Views Counter to the WordPress Dashboard
Displaying the post view count in WordPress dashboard provides a quick overview of your most popular content right away. To do this, add the post view count as a custom column on the All Posts page in your WordPress dashboard.
// Add the custom column "Number of Views" inside the posts page on WP Dashboard
// Add new column to the post list
add_filter( 'manage_posts_columns', 'cdxn_add_views_column' );
function cdxn_add_views_column( $columns ) {
$columns['post_views'] = __( 'Number of Views', 'codexin' );
return $columns;
}
// Render the new custom column
add_action( 'manage_posts_custom_column' , 'cdxn_render_views_column_data', 10, 2 );
function cdxn_render_views_column_data( $column, $post_id ) {
if ( $column === 'post_views' ) {
$post_views = get_post_meta( $post_id, 'cx_post_views', true );
echo esc_html( $post_views ? $post_views : '0' );
}
}
// Add the new column to the post list in a sortable way
add_filter( 'manage_edit-post_sortable_columns', 'cdxn_register_views_column_sortable' );
function cdxn_register_views_column_sortable( $columns ) {
$columns['post_views'] = 'post_views';
return $columns;
}
Now, the “Number of Views” column will appear in the All Posts page of your WordPress dashboard, showing the views count for each post.
In conclusion, having a post views counter is an excellent way to gauge the reach and popularity of your content. Equip yourself with this tutorial to add a post views counter to your WordPress website, understanding your readership’s engagement.
We hope that this tutorial has provided you with valuable insights and made the process straightforward. To further enhance your WordPress experience, here are some additional tips and tricks that can assist you in optimizing and managing your website effectively.