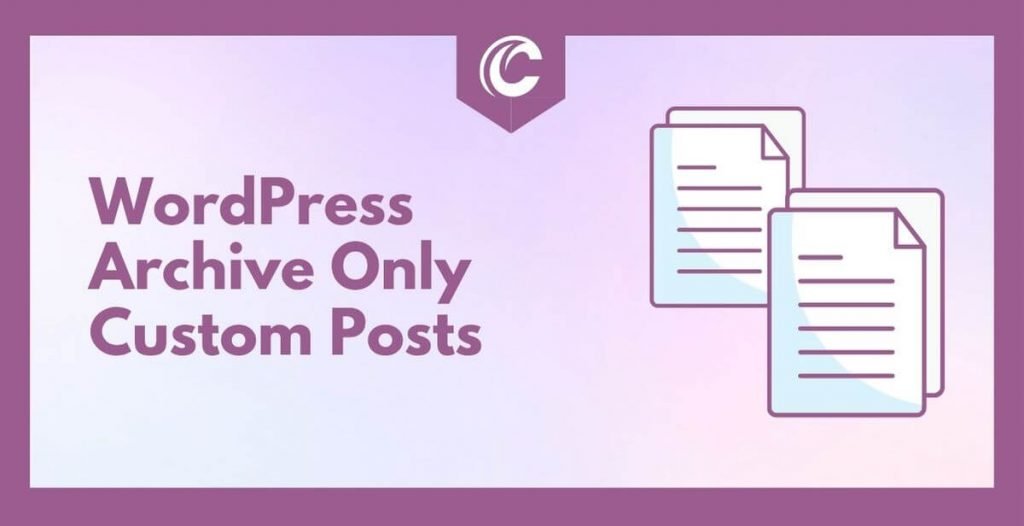
Do you want to know how to create an archive only custom post type in WordPress? WordPress custom post type is a great way of creating different kinds of custom posts, for example, testimonials, teams, doctors, portfolios, etc. A custom post type is almost the same as a regular post type but with a different post_type value in the database. WordPress provides 5 main default posts types for example:
- Posts
- Pages
- Attachments
- Revisions
- Navigation menus
Besides these 5 default post types, you can create additional custom post types based on your requirements. For example, you may want to create “teams” custom post type for the team members of your company. And, each team member may have his/her own photo, job title, job designation, short biography, social profile icons, etc. Using the default posts type, it is troublesome to achieve such requirements. In addition, you also don’t want to take the risk of mixing the regular blog posts’ contents with your team member content. This is the time when you should use a custom post type and custom taxonomies.
What is a WordPress “Archive Only” Custom Post Type?
Every custom post type, by default have two kinds of view.
- Archive view – This is used to show all posts of your custom posts in one location. You should have specific templates for the archive view inside your theme.
- Single View – This is used to show each of the posts individually with all the post details and custom fields specific to that individual post.
And, you are right. “Archive Only” custom post types are those who don’t have a single view page available.
When should you use an “Archive Only” custom post type?
Good question. There are some situations when you don’t want to show a single page for your custom post type. For example, you don’t want to have an individual team member page for all of your team members. You just want the archive page from where visitors can see all team members’ information at a glance.
Another example is, you may want to create a testimonial page from where visitors can see all the testimonials on one page at a glance. You don’t want your visitors to go to each testimonial single page to see that testimonial.
In such situations, it is always good to disable the single page for that custom post type, or in other words, it’s a good practice to create an “Archive Only” custom post type.
How to create an “Archive Only” custom post type?
The process is almost the same as creating a normal custom post type. Here is the trick. After we create the custom post type, we are going to redirect the single view of that custom post type to the archive view.
Let’s just start by creating a Custom Posts type ‘Team’
// register custom 'team' post type
add_action('init', function() {
register_post_type('team',
array(
'labels' => array(
'name' => __( 'Team Members' ),
'singular_name' => __( 'Team Member' ),
'add_new_item' => __('Add New Team Member'),
'edit_item' => __('Edit Member'),
'new_item' => __('New Member'),
'view_item' => __('View Member')
),
'public' => true,
'has_archive' => true,
'publicly_queryable' => true,
)
);
});
After you place this code in your functions.php file, this will create an acrhive page and a single page for this post type. As we want to disable the single view, we will redirect the single page to the Archive page using the template_redirect hook. You can see as per https://codex.wordpress.org:
This action hook executes just before WordPress determines which template page to load. It is a good hook to use if you need to do a redirect with full knowledge of the content that has been queried.
You need to paste the following code to the functions.php
file in your theme.
// redirect single posts to the archive page, scrolled to current ID
add_action( 'template_redirect', function() {
if ( is_singular('team') ) {
global $post;
$redirect_link = get_post_type_archive_link( 'team' );
wp_safe_redirect( $redirect_link, 302 );
exit;
}
});
As you can see, there is a conditional statement is_singular('team')
, this redirection will work only for the single ‘team’ page. The $redirect_link
retrieves the archive URL of ‘team’ and passes the URL to wp_safe_redirect
function. The first parameter wp_safe_redirect()
accepts is the redirection URL. The last parameter is the status code. Default value is 302 which is used for temporary redirection. When this URL is hooking through the template_redirect
the single page will automatically redirect to the Archive page. You can use whatever URL you like to redirect the page. If you need to redirect to the homepage, use home_url()
instead.
In addition, if you don’t need pagination for this specific ‘team’ post, you can use the following code.
// turn off pagination for the archive page
add_action('parse_query', function($query) {
if (is_post_type_archive('team')) {
$query->set('nopaging', 1);
}
});
Action hook and functions references
For a full reference of action hooks and functions used here, see the following:
- template_redirect Action Hook
- get_post_type_archive_link() Function
- wp_safe_redirect() Function
- parse_query Action Hook
- is_post_type_archive() Function
- WP_Query
In conclusion, we hope you found this tutorial to be interesting and informative. If you would like to explore some more tutorials related to WordPress archive post types, please visit these posts
You are also welcome to visit our other blog posts from here related to WordPress.
Thank you